Wake-on-Lan (WoL) in C#
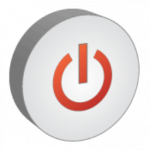
Wake on LAN is a computer networking standard that allows devices to be powered on when they receive a specific network message, if your device supports this feature.
In this example we will demonstrate how to craft this “magic” packet in C#. The concept of the packet is fairly simple. The packet must contain anywhere in the payload six (6) 255 bytes in a row (FF in hexadecimal) followed by the MAC address of the device repeated sixteen times.
As an example, if your device MAC address is 01-00-00-00-00-02 the payload will look like this:
1 | FFFFFFFFFFFF010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002010000000002 |
You can find the implementation of WoL in C# below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | var macAddress = "01-00-00-00-00-02"; // Our device MAC address macAddress = Regex.Replace(macAddress, "[-|:]", ""); // Remove any semicolons or minus characters present in our MAC address var sock = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp) { EnableBroadcast = true }; int payloadIndex = 0; /* The magic packet is a broadcast frame containing anywhere within its payload 6 bytes of all 255 (FF FF FF FF FF FF in hexadecimal), followed by sixteen repetitions of the target computer's 48-bit MAC address, for a total of 102 bytes. */ byte[] payload = new byte[1024]; // Our packet that we will be broadcasting // Add 6 bytes with value 255 (FF) in our payload for (int i = 0; i < 6; i++) { payload[payloadIndex] = 255; payloadIndex++; } // Repeat the device MAC address sixteen times for (int j = 0; j < 16; j++) { for (int k = 0; k < macAddress.Length; k += 2) { var s = macAddress.Substring(k, 2); payload[payloadIndex] = byte.Parse(s, NumberStyles.HexNumber); payloadIndex++; } } sock.SendTo(payload, new IPEndPoint(IPAddress.Parse("255.255.255.255"), 0)); // Broadcast our packet sock.Close(10000); |
Feel free to leave your questions or feedback in the comment section below.
It is not working for me.
This is awesome, and works great. Thank you
You should note that this sends the WoL packet through the default network card, and not all cards if you have more than one.
that very naice thanks